Node, Express, and APIs
Source: An Introduction to Node.js
What is Node.js
- Program to execute JavaScript outside of the browser.
- Built on V8 engine– open source JS engine runs runs in Chromium based browsers.
Installing Node.js
- Direct from node download page
- (Preferred) Using a version manager, NVM, so you can have multiple versions of Node at once.
-
To check version install
-
To run js file in node
Package Management w/ NPM
- Node is bundled with a package manager, NPM
- NPM is the JS package manger, and largest registry
-
To check version install
To install a package globally
To install a package locally
npm init -y
npm install lodash --save
This process does 2 things:
- Creates a package.json file in the directory
- Package.json file describes dependencies, allowing other devs to use NPM to install them
- Includes a node_modules folder with relevant files.
- Don’t include in version control.
const _ = require('lodash');
This specifies which packages are neded in a file
Using Node.js
- Many uses: bundling files and dependencies, running tests, code linting, style checking
Run JavaScript on the Server
The biggest use case for Node.js
- Node.js is single threaded and event driven
- Allows non-blocking processing of actions
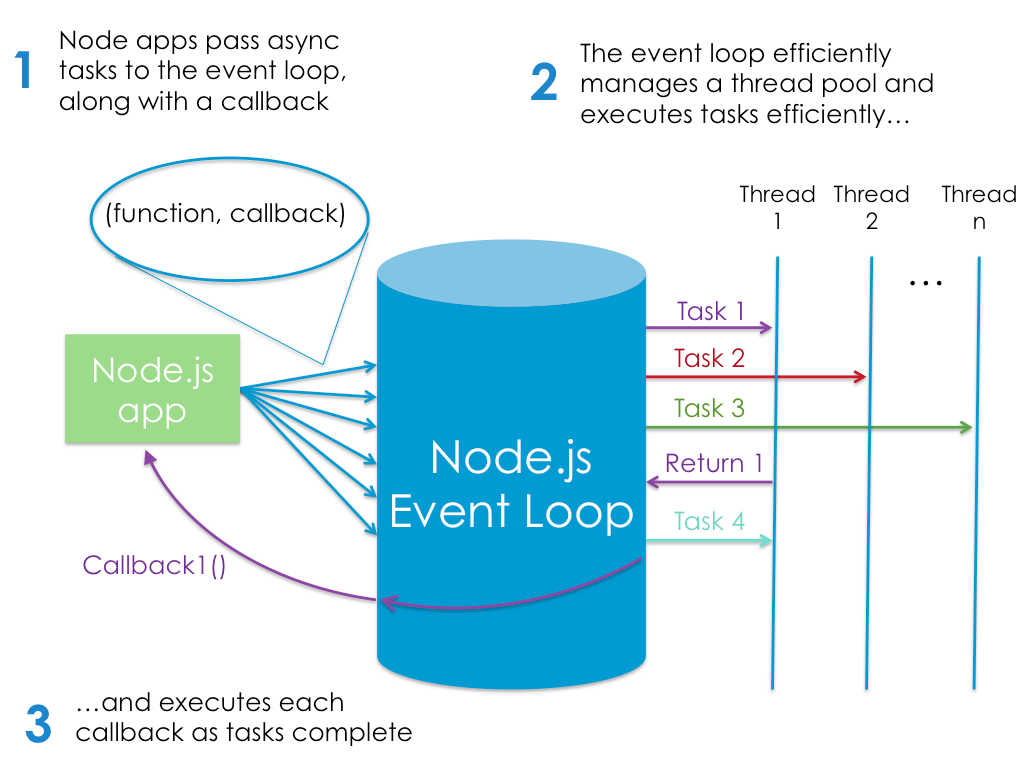
Example
hello-world-server.js
const http = require('http');
http.createServer((request, response) => {
response.writeHead(200);
response.end('Hello, World!');
}).listen(3000);
console.log('Server running on http://localhost:3000');
node hello-world-server.js
Browser: http://localhost:3000
Note: Use nodemon to autoreload server changes
Disadvantages
- Since Node is single threaded, avoid blocking I/O calls.
- Hand off CPU-intensive operations to worker thread
Advantages
- Speed / scalability
- Use same language for backend / frontend
- JSON (same syntax browser <-> server <-> client)
Use Node.js for…
- Real time interactions
- APIs
- Data streaming
Other Uses
- Scripting
- Cross-platform desktop apps